Gin Rummy#
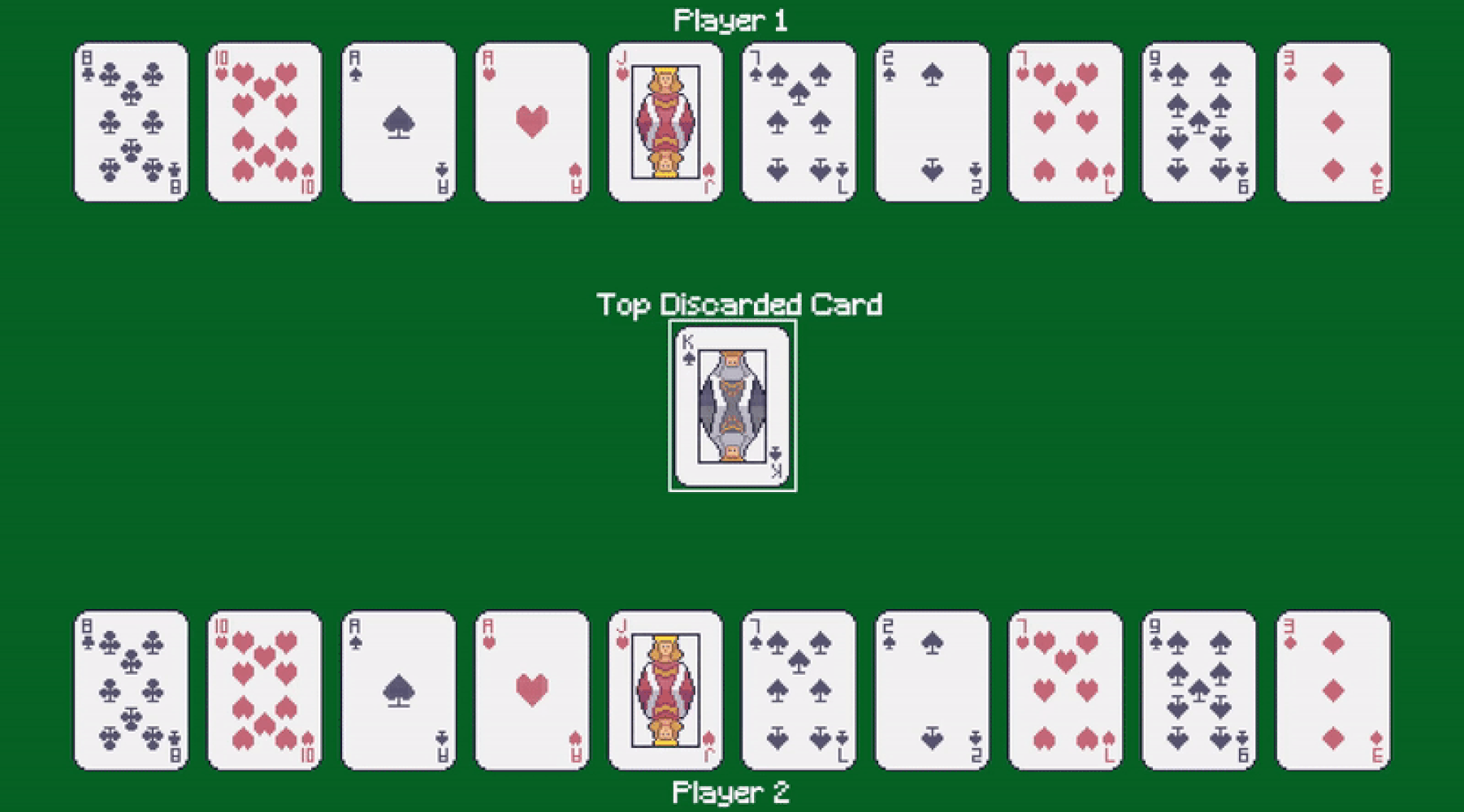
This environment is part of the classic environments. Please read that page first for general information.
Import |
|
---|---|
Actions |
Discrete |
Parallel API |
Yes |
Manual Control |
No |
Agents |
|
Agents |
2 |
Action Shape |
Discrete(110) |
Action Values |
Discrete(110) |
Observation Shape |
(5, 52) |
Observation Values |
[0,1] |
Gin Rummy is a 2-player card game with a 52 card deck. The objective is to combine 3 or more cards of the same rank or in a sequence of the same suit.
Our implementation wraps RLCard and you can refer to its documentation for additional details. Please cite their work if you use this game in research.
Arguments#
Gin Rummy takes two optional arguments that define the reward received by a player who knocks or goes gin. The default values for the knock reward and gin reward are 0.5 and 1.0, respectively.
gin_rummy_v4.env(knock_reward = 0.5, gin_reward = 1.0, opponents_hand_visible = False)
knock_reward
: reward received by a player who knocks
gin_reward
: reward received by a player who goes gin
opponents_hand_visible
: Set to True
to observe the entire observation space as described in Observation Space
below. Setting it to False
will remove any observation of the unknown cards and the observation space will only include planes 0 to 3.
Observation Space#
The observation is a dictionary which contains an 'observation'
element which is the usual RL observation described below, and an 'action_mask'
which holds the legal moves, described in the Legal Actions Mask section.
The main observation space is 5x52 with the rows representing different planes and columns representing the 52 cards in a deck. The cards are ordered by suit (spades, hearts, diamonds, then clubs) and within each suit are ordered by rank (from Ace to King).
Row Index |
Description |
---|---|
0 |
Current player’s hand |
1 |
Top card of the discard pile |
2 |
Cards in discard pile (excluding the top card) |
3 |
Opponent’s known cards |
4 |
Unknown cards |
Column Index |
Description |
---|---|
0 - 12 |
Spades |
13 - 25 |
Hearts |
26 - 38 |
Diamonds |
39 - 51 |
Clubs |
Legal Actions Mask#
The legal moves available to the current agent are found in the action_mask
element of the dictionary observation. The action_mask
is a binary vector where each index of the vector represents whether the action is legal or not. The action_mask
will be all zeros for any agent except the one
whose turn it is. Taking an illegal move ends the game with a reward of -1 for the illegally moving agent and a reward of 0 for all other agents.
Action Space#
There are 110 actions in Gin Rummy.
Action ID |
Action |
---|---|
0 |
Score Player 0 |
1 |
Score Player 1 |
2 |
Draw a card |
3 |
Pick top card from Discard pile |
4 |
Declare dead hand |
5 |
Gin |
6 - 57 |
Discard a card |
58 - 109 |
Knock |
For example, you would use action 2
to draw a card or action 3
to pick up a discarded card.
Rewards#
At the end of the game, a player who gins is awarded 1 point, a player who knocks is awarded 0.5 points, and the losing player receives a reward equal to the negative of their deadwood count.
If the hand is declared dead, both players get a reward equal to negative of their deadwood count.
End Action |
Winner |
Loser |
---|---|---|
Dead Hand |
– |
|
Knock |
– |
|
Gin |
– |
|
Note that the defaults are slightly different from those in RLcard- their default reward for knocking is 0.2.
Penalties of deadwood_count / 100
ensure that the reward never goes below -1.
Version History#
v4: Upgrade to RLCard 1.0.3 (1.11.0)
v3: Fixed bug in arbitrary calls to observe() (1.8.0)
v2: Bumped RLCard version, bug fixes, legal action mask in observation replaced illegal move list in infos (1.5.0)
v1: Bumped RLCard version, fixed observation space, adopted new agent iteration scheme where all agents are iterated over after they are done (1.4.0)
v0: Initial versions release (1.0.0)
Usage#
AEC#
from pettingzoo.classic import gin_rummy_v4
env = gin_rummy_v4.env(render_mode="human")
env.reset(seed=42)
for agent in env.agent_iter():
observation, reward, termination, truncation, info = env.last()
if termination or truncation:
action = None
else:
mask = observation["action_mask"]
# this is where you would insert your policy
action = env.action_space(agent).sample(mask)
env.step(action)
env.close()
API#
- class pettingzoo.classic.rlcard_envs.gin_rummy.raw_env(knock_reward: float = 0.5, gin_reward: float = 1.0, opponents_hand_visible: bool | None = False, render_mode: str | None = None, screen_height: int | None = 1000)[source]#
- observe(agent)[source]#
Returns the observation an agent currently can make.
last() calls this function.
- render()[source]#
Renders the environment as specified by self.render_mode.
Render mode can be human to display a window. Other render modes in the default environments are ‘rgb_array’ which returns a numpy array and is supported by all environments outside of classic, and ‘ansi’ which returns the strings printed (specific to classic environments).