Combat: Tank¶
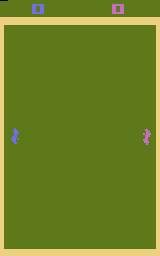
This environment is part of the Atari environments. Please read that page first for general information.
Import |
|
---|---|
Actions |
Discrete |
Parallel API |
Yes |
Manual Control |
No |
Agents |
|
Agents |
2 |
Action Shape |
(1,) |
Action Values |
[0,5] |
Observation Shape |
(210, 160, 3) |
Observation Values |
(0,255) |
Combat’s classic tank mode is an adversarial game where prediction, and positioning are key.
The players move around the map. When your opponent is hit by your bullet, you score a point. Note that your opponent gets blasted through obstacles when it is hit, potentially putting it in a good position to hit you back.
Whenever you score a point, you are rewarded +1 and your opponent is penalized -1.
Environment parameters¶
Some environment parameters are common to all Atari environments and are described in the base Atari documentation.
Parameters specific to combat-tank are
combat_tank_v2.env(has_maze=True, is_invisible=False, billiard_hit=True)
has_maze
: Set to true to have the map be a maze instead of an open field
is_invisible
: If true, tanks are invisible unless they are firing or are running into a wall.
billiard_hit
: If true, bullets bounce off walls, in fact, like billiards, they only count if they hit the opponent’s tank after bouncing off a wall.
Action Space¶
In any given turn, an agent can choose from one of 18 actions.
Action |
Behavior |
---|---|
0 |
No operation |
1 |
Fire |
2 |
Move up |
3 |
Move right |
4 |
Move left |
5 |
Move down |
6 |
Move upright |
7 |
Move upleft |
8 |
Move downright |
9 |
Move downleft |
10 |
Fire up |
11 |
Fire right |
12 |
Fire left |
13 |
Fire down |
14 |
Fire upright |
15 |
Fire upleft |
16 |
Fire downright |
17 |
Fire downleft |
Version History¶
v2: Minimal Action Space (1.18.0)
v1: Breaking changes to entire API (1.4.0)
v0: Initial versions release (1.0.0)
Usage¶
AEC¶
from pettingzoo.atari import combat_tank_v2
env = combat_tank_v2.env(render_mode="human")
env.reset(seed=42)
for agent in env.agent_iter():
observation, reward, termination, truncation, info = env.last()
if termination or truncation:
action = None
else:
# this is where you would insert your policy
action = env.action_space(agent).sample()
env.step(action)
env.close()
Parallel¶
from pettingzoo.atari import combat_tank_v2
env = combat_tank_v2.parallel_env(render_mode="human")
observations, infos = env.reset()
while env.agents:
# this is where you would insert your policy
actions = {agent: env.action_space(agent).sample() for agent in env.agents}
observations, rewards, terminations, truncations, infos = env.step(actions)
env.close()