Space Invaders¶
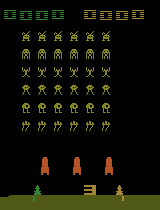
This environment is part of the Atari environments. Please read that page first for general information.
Import |
|
---|---|
Actions |
Discrete |
Parallel API |
Yes |
Manual Control |
No |
Agents |
|
Agents |
2 |
Action Shape |
(1,) |
Action Values |
[0,5] |
Observation Shape |
(210, 160, 3) |
Observation Values |
(0,255) |
Classic Atari game, but there are two ships controlled by two players that are each trying to maximize their score.
This game has a cooperative aspect where the players can choose to maximize their score by working together to clear the levels. The normal aliens are 5-30 points, depending on how high up they start, and the ship that flies across the top of the screen is worth 100 points.
However, there is also a competitive aspect where a player receives a 200 point bonus when the other player is hit by the aliens. So sabotaging the other player somehow is a possible strategy.
The number of lives is shared between the ships, i.e. the game ends when a ship has been hit 3 times.
Official Space Invaders manual
Environment parameters¶
Some environment parameters are common to all Atari environments and are described in the base Atari documentation.
Parameters specific to Space Invaders are
space_invaders_v2.env(alternating_control=False, moving_shields=True,
zigzaging_bombs=False, fast_bomb=False, invisible_invaders=False)
alternating_control
: Only one of the two players has an option to fire at one time. If you fire, your opponent can then fire. However, you can’t hoard the firing ability forever, eventually, control shifts to your opponent anyways.
moving_shields
: The shields move back and forth, leaving less reliable protection.
zigzaging_bombs
: The invader’s bombs move back and forth, making them more difficult to avoid.
fast_bomb
: The bombs are much faster, making them more difficult to avoid.
invisible_invaders
: The invaders are invisible, making them more difficult to hit.
Action Space (Minimal)¶
In any given turn, an agent can choose from one of 6 actions.
Action |
Behavior |
---|---|
0 |
No operation |
1 |
Fire |
2 |
Move up |
3 |
Move right |
4 |
Move left |
5 |
Move down |
Version History¶
v2: Minimal Action Space (1.18.0)
v1: Breaking changes to entire API (1.4.0)
v0: Initial versions release (1.0.0)
Usage¶
AEC¶
from pettingzoo.atari import space_invaders_v2
env = space_invaders_v2.env(render_mode="human")
env.reset(seed=42)
for agent in env.agent_iter():
observation, reward, termination, truncation, info = env.last()
if termination or truncation:
action = None
else:
# this is where you would insert your policy
action = env.action_space(agent).sample()
env.step(action)
env.close()
Parallel¶
from pettingzoo.atari import space_invaders_v2
env = space_invaders_v2.parallel_env(render_mode="human")
observations, infos = env.reset()
while env.agents:
# this is where you would insert your policy
actions = {agent: env.action_space(agent).sample() for agent in env.agents}
observations, rewards, terminations, truncations, infos = env.step(actions)
env.close()